Thursday, December 14, 2006
Tuesday, October 31, 2006
This is for version 3.2.1 Release (Build 324)
I tried the wget approach, no luck :(
I tried the php approach (btw typo for C:\php\php.exe C:\wwwroot\inetpub\cerberus-gui\cron.php ) the path should be c:\inetpub\wwwroot\) but I would always get, this IP is not authorized to run the schedule, even though I had added 127.0.0.1, tried other masks etc..) :(
So.. the way I finally did get it to work is using the windows port of lynx from here http://www.pervalidus.net/cygwin/lynx/ (at the bottom)
I set up a windows task using "C:\Program Files\lynx\lynx.exe" --dump http://localhost/cerberus-gui/cron.php?verbose=1
with a start in: "c:\program files\lynx\"
You also of course need to set it to external timer in configuration -> scheduled tasks and you also need to add the getmail scheduled task and make sure it is enabled.
Hope this helps someone else out there!
Saturday, October 21, 2006
Tuesday, October 17, 2006
Friday, October 13, 2006
http://www.daniweb.com/code/snippet451.html
# retrieve the file information from a selected folder
# sort the files by last modified date/time and display in order newest file first
# tested with Python24 vegaseat 21jan2006
import os, glob, time
# use a folder you have ...
root = 'c:\\test\\' # one specific folder
#root = 'D:\\Zz1\\*' # all the subfolders too
print '-'*60 # just vanity
date_file_list = []
for folder in glob.glob(root):
print "folder =", folder
# select the type of file, for instance *.jpg or all files *.*
for file in glob.glob(folder + '/*.*'):
# retrieves the stats for the current file as a tuple
# (mode, ino, dev, nlink, uid, gid, size, atime, mtime, ctime)
# the tuple element mtime at index 8 is the last-modified-date
stats = os.stat(file)
# create tuple (year yyyy, month(1-12), day(1-31), hour(0-23), minute(0-59), second(0-59),
# weekday(0-6, 0 is monday), Julian day(1-366), daylight flag(-1,0 or 1)) from seconds since epoch
# note: this tuple can be sorted properly by date and time
creation_date = time.localtime(stats[9])
#print image_file, lastmod_date # test
# create list of tuples ready for sorting by date
date_file_tuple = creation_date, file
date_file_list.append(date_file_tuple)
#print date_file_list # test
date_file_list.sort()
date_file_list.reverse() # newest mod date now first
print "%-40s %s" % ("filename:", "Creation Date:")
for file in date_file_list:
# extract just the filename
folder, file_name = os.path.split(file[1])
# convert date tuple to MM/DD/YYYY HH:MM:SS format
file_date = time.strftime("%m_%d_%y", file[0])
year_date = time.strftime("%Y", file[0])
month_date = time.strftime("%m", file[0])
day_date = time.strftime("%d", file[0])
print "%-40s %s" % (file[1], file_date)
print year_date
print month_date
print day_date
DateDir = 'c:\\DateTest\\'+ year_date + '\\' + month_date + '\\' + day_date
if not os.path.isdir(DateDir): #check whether the dir exists, if not create it
os.makedirs(DateDir)
print "xcopy /Y /F " + '"' + file[1] + '" ' + DateDir
os.popen("move /Y " + '"' + file[1] + '" ' + DateDir).read()
from time import gmtime, strftime
#Get yesterdays date and time (from http://www.thejackol.com/2005/07/01/yesterdays-date-in-python/)
now = time.time()
today = time.localtime(now)
yesterday = strftime('"%a-%m/%d/%Y"',time.localtime(now - 60*60*24))
or
import datetime
now = datetime.datetime.now()
one_day = datetime.timedelta(hours=24)
yesterday = now - one_day
print strftime('"%a-%m/%d/%Y"',yesterday.timetuple())
Tuesday, September 26, 2006
zip = zipfile.ZipFile('/afile.zip', 'w', zipfile.ZIP_DEFLATED)
zipdir('/adirectory/', zip)
zip.close()
I couldn't get this to work unless I specified zipfile.ZIP_DEFLATED of course after reading I found out the default is zipfile.ZIP_STORED. If you don't specify ZIP_DEFLATED it creates a zip file that has 0% compression (basically just stores it) go figure :-)
Another thing I kept banging my head against was how to hide a dos popup window from a py2exe created file. A combination of
os.popen("xcopy /Y /F " + ServerPath + " c:\\").read()
PLUS in the setup file for py2exe change setup(console=['whatever.pyw']) to setup(windows=['whatever.pyw'])
Cool stuff this python
Tuesday, September 19, 2006
Metadot: Setup LDAP authentication for active directory on server 2003
Under manage/config/
Click enable for LDAP registration
Click Modify under registration params
LDAP server should be your LDAP server, either name or IP
BASE DN:
This is where the LDAP searches will begin
In my environment it is
OU=National Users,DC=nffc,DC=local
One way you can find this out is by using a program called OldCmp http://www.joeware.net/win/free/tools/oldcmp.htm
Run this command oldcmp -report -age 1 -users (This generates a report of users passwords that are older then 1 day)
Open this report and look at your users, it should help in figuring out what your base DN is.
Next you need the user unique identifier, this was confusing for a bit, but this is the filed that LDAP is going to look up against. (Which is also why you can assign it a label in case your want to compare on something different then email address)
A couple of 'User unique identifier in LDAP directory options' are: Mail, userPrincipalName or CN more info http://www.computerperformance.co.uk/Logon/LDAP_attributes_active_directory.htm
Mail ---> The users email address
userPrincipalName ---> The users logon on name i.e. jschmoe@mydomain.local
CN ---> The users name i.e. joe schmoe
I configured it to use LDAP for authentication only so I have not messed with profile management yet.
The last hoop to configure is click the radial button to select DN: (you have to do this for active directory)
Now you need to supply a user that can do LDAP lookups. After you create this user you can use OldCmp to find out its DN
An example DN is cn=metadot,ou=service accounts,ou=national users,dc=nffc,dc=local
and then of course set the password.
Tuesday, June 20, 2006
Friday, June 09, 2006
Tuesday, May 16, 2006
Wednesday, May 10, 2006
Tuesday, May 09, 2006
Setting up auto enrollment for email certificates using active directory
We must issue a Key Recovery Agent certificate for this user. To do this:
Issue a new template named Key Recovery Agent
Request this certificate for the user who becomes the Key Recovery Agent
Manually Issue the Key Recovery Agent Certificate at the CA
Figure 1 shows the issued Key Recovery Agent Certificate for the user Administrator.
Figure 1: Issued Key Recovery Agent certificate
Important:
The Windows 2003 CA will not automatically issue this certificate to the user who requests the Key Recovery Agent certificate. The CA Administrator must manually Issue the certificate in the Microsoft CA
Now it is time to enable the CA for Key Archiving. Start the Microsoft CA
Now you have to configure the following settings for this template: (I also checked do not automatically reenroll if a duplicate certificate exists, this will keep someone from logging on a different computer and receiving a new cert and getting their mail all messed up because they would have incompatible keys and their email would get encrypted with the wrong ones possibly.
Publishing all certificates in Active Directory is required, because the “Global Address List” of Exchange Server 2003 is based on AD. If you have configured this feature, all certificates are available in Active Directory.
In “Request Handling Properties” you have to choose signature and encryption and you should configure key archiving to provide key recovery. In addition to this, enrolling the certificate without requiring user input is the proposed solution.
To make sure that the old Exchange User template would never be used again you should configure it so that this new template supersedes the old one.
I created a group called email encryption that I then added members to provide them with certs.
Next you want to set up group policy to allow auto-enrollment
Configuring Certificate Services for Autoenrollment
Autoenrollment is a useful feature of certification services in Windows XP and Windows Server 2003, Standard Edition. Autoenrollment allows the administrator to configure subjects to automatically enroll for certificates, retrieve issued certificates, and renew expiring certificates without requiring subject interaction. The subject does not need to be aware of any certificate operations, unless you configure the certificate template to interact with the subject.
Wednesday, May 03, 2006
We will set this class to sort by the FullName property in ascending order.
Code:
Public Function CompareTo(ByVal obj As Object) As Integer Implements System.IComparable.CompareTo
Dim p As Person
' Any non-Nothing object is greater than nothing.
If obj Is Nothing Then
Return 1
End If
' Avoid late-binding and cast to a specific Person Object.
p = CType(obj, Person)
' Use the String's CompareTo Method to perform the check.
Return Me.FullName.CompareTo(p.FullName)
End Function
Tuesday, May 02, 2006
Monday, May 01, 2006
Friday, April 28, 2006
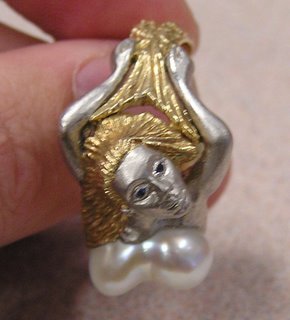
So I'm engaged, did you know that? Probably you do since nobody else besides family reads this blog :-) anyways so spent last night looking at rings (and other jewelry) that Grandpa Nissan created. It is truly amazing work. So today I followed a reference of his to downtown Seattle to look for some diamonds to put in the ring that Heidi picked out. They remembered him there and also returned some jewelry of his, which is why I have a rather busty looking mermaid ring in my pocket right now... it looks like this
Thursday, April 27, 2006
First get yourself an account at https://www.cacert.org this place is awesome since I detest paying for anything... especially a cert.
I use this same account for multiple people in the office. Let's say I'm settting up joe@joe.com I first click add under email accounts and then type in joe@joe.com. This then sends Joe an email with the subject [Cacert.org] Mail Probe and a link that he must click in order to verify his address.
After he has clicked on the link I log back into cacert.org and then click Client Certificates/New. I then create a new certificate using joe@joe.com. I use the default of (Microsofot Enhanced Cryptographic Provider v1.0) and Install the Certificate.
Once the cert is installed I export it with the private key so I can send it to joe, and I export the public key so I can publish to GAL using active directory.
This is accomplished by opening IE, going to tools, Internet options, content tab, Certificates button, under personal tab I click on the cert I want to export and then click export. I choose to export the private key and I export Joe.pfx
I repeat this process but this time I only export the public key saving it as Joe.cer
***This part is sneaky vodoo***! (Hard to find instructions for this on the net)
Next I go to the email server and publish the Joe.cer (public cert).
First copy Joe.cer over to the email server. Then open the exhange system manager on the email server. Then, recipients, All global Address Lists, R-click on Default Global Address List, Properties, And then (drum roll please) hit the preview button, then find and double click the person you want to add a cert to, then go to published certificates tab, then click add from file. This will publish their cert to the GAL (global address list)
Next I like to send them a test encrypted message. Outlook is funny you have to hit the options button (this is also under view, options) and then enable sign and encrypt.
Next we need to enable joe to be able to actually read his encrypted email. To do this send joe his joe.pfx and have him import it. That should do it.